rong bài viết này, chúng ta sẽ tìm hiểu cách phát hiện xe máy trong video sử dụng mô hình YOLOv8. YOLO (You Only Look Once) là một trong những công nghệ phát hiện và nhận diện đối tượng nhanh nhất hiện nay, phù hợp để ứng dụng trong nhiều tình huống thực tế như giao thông, giám sát, và ô tô tự hành.
Tóm Tắt
- Ngôn ngữ: Python
- Thư viện: OpenCV, PyTorch, YOLOv8
- Kết quả: Nhận diện xe máy trong video theo thời gian thực và hiển thị kết quả.
1. Yêu Cầu Chuẩn Bị
Các công cụ cần thiết:
- Python đã được cài đặt (phiên bản >= 3.8).
- Thư viện OpenCV để xử lý video.
- Thư viện PyTorch để load mô hình.
- Thư viện “Ultralytics” dùng cho YOLOv8.
Cài đặt thư viện:
Chạy lệnh sau trong terminal để cài đặt các thư viện cần thiết:
pip install opencv-python torch ultralytics
2. Kết Quả Minh Họa
Trước khi xem mã nguồn, hãy cùng xem kết quả mô phỏng nhận diện xe máy trong video.
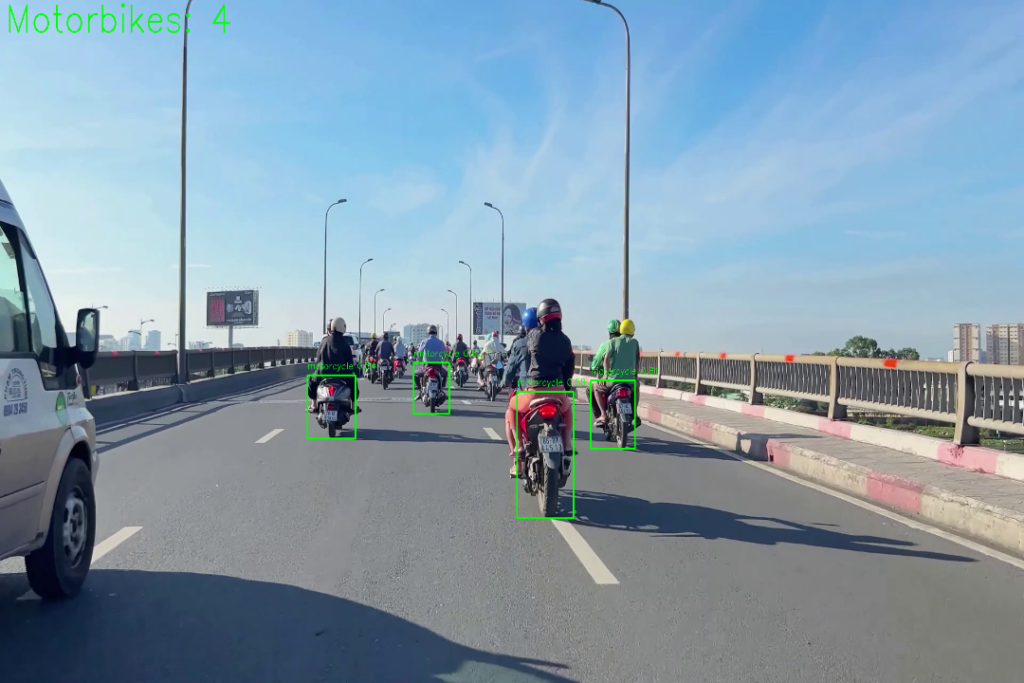
3. Mã Nguồn Chi Tiết
3.1. Tải mô hình YOLO
from ultralytics import YOLO
def load_model(model_path):
"""
Tải mô hình YOLO từ đường dẫn chỉ định.
"""
return YOLO(model_path)
3.2. Xử lý Từng Frame Video
import cv2
def process_frame(frame, model, confidence_threshold):
"""
Xử lý một frame và phát hiện xe máy bằng mô hình YOLO.
Args:
frame (ndarray): Frame cần xử lý.
model (YOLO): Mô hình YOLO để phát hiện.
confidence_threshold (float): Ngưỡng độ tin cậy để lọc kết quả.
Returns:
tuple: Frame đã được xử lý, số lượng xe máy phát hiện được.
"""
results = model(frame)
motorbike_count = 0
font = cv2.FONT_HERSHEY_SIMPLEX
font_scale = 0.5
font_color = (0, 255, 0)
thickness = 1
for result in results:
boxes = result.boxes # Hộp giới hạn (bounding boxes)
for box in boxes:
x1, y1, x2, y2 = map(int, box.xyxy[0])
class_id = int(box.cls[0])
confidence = float(box.conf[0])
class_name = model.names[class_id]
if class_name.lower() == 'motorcycle' and confidence >= confidence_threshold:
motorbike_count += 1
label = f"{class_name} {confidence:.2f}"
cv2.rectangle(frame, (x1, y1), (x2, y2), (0, 255, 0), 2)
cv2.putText(frame, label, (x1, y1 - 10), font, font_scale, font_color, thickness)
return frame, motorbike_count
3.3. Hiển Thị Video Kèm Phát Hiện
import cv2
def display_video(video_path, model, confidence_threshold):
"""
Hiển thị video kèm phát hiện xe máy và đếm theo thời gian thực.
Args:
video_path (str): Đường dẫn tới file video.
model (YOLO): Mô hình YOLO để phát hiện đối tượng.
confidence_threshold (float): Ngưỡng độ tin cậy để lọc kết quả.
"""
cap = cv2.VideoCapture(video_path)
if not cap.isOpened():
print("Lỗi: Không thể mở file video.")
return
font = cv2.FONT_HERSHEY_SIMPLEX
font_scale = 2
font_color = (0, 255, 0)
thickness = 2
while True:
ret, frame = cap.read()
if not ret:
break
frame, motorbike_count = process_frame(frame, model, confidence_threshold)
cv2.putText(frame, f"Motorbikes: {motorbike_count}", (10, 50), font, font_scale, font_color, thickness)
output_width, output_height = 1080, 720
frame = cv2.resize(frame, (output_width, output_height))
cv2.imshow('YOLOv8 Detection', frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
3.4. Kết Nối Camera IP RTSP
Nếu bạn muốn phát hiện xe máy trong video trực tiếp từ camera IP, hãy thay đổi video_path
bằng URL RTSP của camera:
rtsp_url = 'rtsp://username:password@<ip_address>:<port>/path'
def display_rtsp(rtsp_url, model, confidence_threshold):
"""
Hiển thị video trực tiếp từ camera IP RTSP và phát hiện xe máy.
Args:
rtsp_url (str): URL RTSP của camera IP.
model (YOLO): Mô hình YOLO để phát hiện đối tượng.
confidence_threshold (float): Ngưỡng độ tin cậy để lọc kết quả.
"""
cap = cv2.VideoCapture(rtsp_url)
if not cap.isOpened():
print("Lỗi: Không thể kết nối tới camera IP.")
return
font = cv2.FONT_HERSHEY_SIMPLEX
font_scale = 2
font_color = (0, 255, 0)
thickness = 2
while True:
ret, frame = cap.read()
if not ret:
break
frame, motorbike_count = process_frame(frame, model, confidence_threshold)
cv2.putText(frame, f"Motorbikes: {motorbike_count}", (10, 50), font, font_scale, font_color, thickness)
output_width, output_height = 1080, 720
frame = cv2.resize(frame, (output_width, output_height))
cv2.imshow('YOLOv8 RTSP Detection', frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
3.5. Hàm Chính Chạy Chương Trình
Sử dụng video
if __name__ == "__main__":
model_path = '{File}.pt' # Lấy từ model yolo hoặc tự train
video_path = '{File}.mp4' # file video
confidence_threshold = 0.3
print("Đang tải mô hình YOLO...")
model = load_model(model_path)
print("Bắt đầu phát hiện video...")
display_video(video_path, model, confidence_threshold)
Sử dụng Camera IP
if __name__ == "__main__":
model_path = 'model/yolov8x.pt'
rtsp_url = 'rtsp://username:password@<ip_address>:<port>/path'
confidence_threshold = 0.3
print("Đang tải mô hình YOLO...")
model = load_model(model_path)
print("Bắt đầu phát hiện video RTSP...")
display_rtsp(rtsp_url, model, confidence_threshold)
4. Kết Luận
Với YOLOv8, bạn có thể nhanh chóng phát triển một chương trình phát hiện xe máy trong video, phục vụ cho nhiều ứng dụng thực tế. Để minh hoạ, hãy chia sẻ trải nghiệm của bạn với chúng tôi tại TechSphereX!
Video demo